Uploader
The wm-uploader
component is to be used in situations where users are uploading files that they will be able to access later; it is not for entering data into our system, for example by uploading a CSV. At this point, the component does not have filtering capabilities; if the pattern you are implementing includes a filter section, you won't be able to use the component.
The uploader comprises the input and the file list. It takes a list of files to display as children wm-file
elements. When the user selects files, the component exposes the files to upload via the wmUploaderFilesSelected
event. The files can then be validated, uploaded, and added as children. When the user clicks on a File's preview, delete, or download button, an event is emitted so that you can create a callback function to handle these cases (see API tab).
File List Component for use cases where a read-only list of files is needed and no other files need to be uploaded.
Use the
wm-uploader
and ensure proper screen reader accessibility, wm-file
progress
must be kept up to date. See the Properties tab for more information.
* Required property
string
string
required-field
error
"Please add at least one file".
string
pdf txt log xml doc docx xls xlsx ppt pptx gif jpg png csv
. Note: Validation and error message
setting should be handled outside of the component.
string
f1c0
).string
string
100 MB
. Note: Validation and error
message setting should be handled outside of the component.
number
boolean
label
property.
time | size | none
time
.
Properties of the children wm-file
.
string
string
string
pdf
, not application/pdf
.
string
string
preview download delete
. Defaults to
download delete
.
ISO string
2020-01-28T22:29:10.397Z
string
number
Please note that the examples below do not actually upload a file to a server.
Usage in HTML
Edit the code below to see changes reflected in the example above.
Javascript Sample
Example code for functionality.
Usage in Elm
Code generated from HTML.
wmFilesSelected
.
Properties of the children wm-file
.
The number of files uploading is shown as a notification and announced via an aria-live region.
When a file is deleted, you should announce it with the snackbar component.
Example
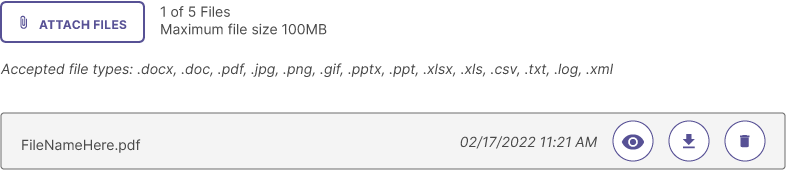
Anatomy
The Uploader component consists of a secondary button with an icon, number of attachments that can be uploaded as well as maximum file size, and helper text of the accepted file types.

- Button - The button is a secondary button with an icon. Language for the button should use the same words that a descriptor might have. For example, If the instructions say, “Attach important reports and documents that demonstrate your organization’s value and design, as well as evidence of success and improvement”, then you would use the language “Attach Files” in your button. Always pair the text with an icon that is appropriate with the language.
- Accepted file types - List the files accepted by the uploader by type. For example, group the image types together, then documents, then videos.
- Number of attachments (optional) - To the right of the button, you can include a label that says “Attach up to ___ files”. When the number of files have reached the maximum amount, change the language to say “Maximum number of files reached”
Tip: Make sure the language is consistent with the button language. - Maximum file size - To the right of the upload button, the maximum file size automatically shows if the property is set on the component by the developer. By default, it is 100MB, but may change depending on the use case.
Once a file is uploaded, a file block is added below it.

- File name - The file name is always present and has the file type at the end of it.
- Date uploaded / File size (optional) - Only one of these tags should be present. The “date uploaded” or “file size” tag is right-aligned and pinned to the buttons on the right. If there is a change in the number of buttons, the text should move to the right and stay pinned to the button. The date format should always be MM/DD/YYYY and keep with 12 hour time, and file size format should always be ##MB (or KB, or GB, depending on the size. The date or file size tag is not present in certain use cases where it is not beneficial to the user. The date or file size tag is also not present when the screen size is in a mobile format.
- Preview button (optional) - If the use case calls for it, use a preview button. If preview is to be used, it is the first icon button shown.
- Download button (optional) - If the use case calls for it, use a download button. Download comes before delete, but after preview (if used), and can also be used on its own.
- Delete button (optional) - If the permissions for the user allow it, use the delete button. If it’s grouped with other buttons, it is the last icon button shown. It can also be used on its own. Once clicked, it will trigger a snackbar to alert the user that the file has been deleted.
States
Empty
When no files have been uploaded.

New file uploaded
When a new file is uploaded, a check mark appears showing that the upload was successful. The checkbox persists for the duration of the session.

File uploaded
The label for how many files have been uploaded changes based on the amount of files that have been uploaded.
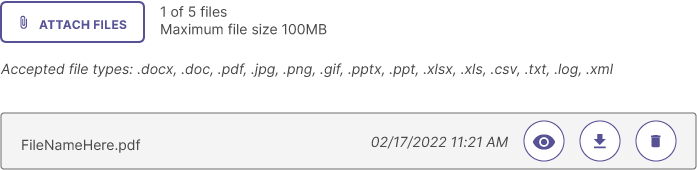
File block content combinations
The file block is flexible so that you can use three different buttons in different combinations or not at all, depending on what the use case is. Keep in mind that everything is optional EXCEPT for the file name itself. Think about the use case and what actions the user needs to make and what information is necessary for that particular user.





Error
If the user is trying to upload a file that is not a supported file type, or if the file is too big, an error is displayed. The user will have the ability to clear each error.

If the user has selected mulitple files at a time to upload, but that number exceeds the limit, an error will appear stating that the upload was unsuccessful.
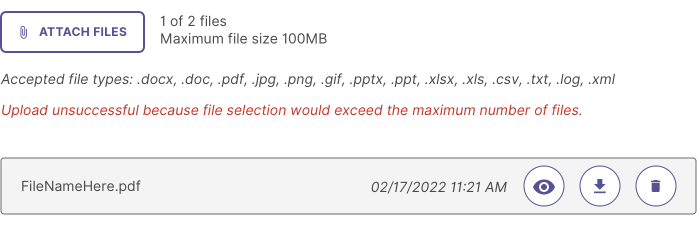
Required State
Use a label with an asterisk to indicate that a file is required. The label’s action verb should match the verb used in the button, i.e. “Attach your Capstone Files” with the button “Attach Files”, or “Upload Program Files” with the button “Upload Files”.

If no file has been added, an error state is displayed and the user will be prevented from moving on until a file is added.

Smaller screen size
When the uploader is smaller than 500px, a truncated view of the file block is shown. If there are two or more buttons needed for the file block, the buttons are wrapped in an Action Menu. If there is only one button, there is no need to change or remove any buttons. At this size, the date or file size are not shown.

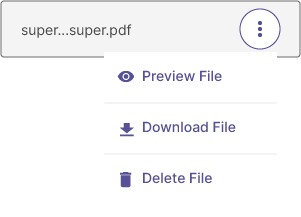
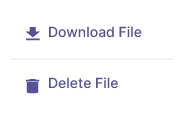
Tip: Make sure that the overflow menu items are in the same order as the buttons it is replacing.

Max width
The max width of the file block is 1140px.
File uploading
During the upload, the file block displays a progress bar at the top. Only the file name is displayed.
Once the file is uploaded, the user will see the green check mark as well as the date uploaded and any file block buttons.

Button behavior
Attach files button
The button type (secondary with an icon) will always be the same for the uploader. It must contain an icon and supporting button text.
Action buttons
Once files have been uploaded, the file blocks contain a few different action buttons to choose from.
Spacing
Pattern Spacing
Pattern spacing is built into the component and should not be adjusted.
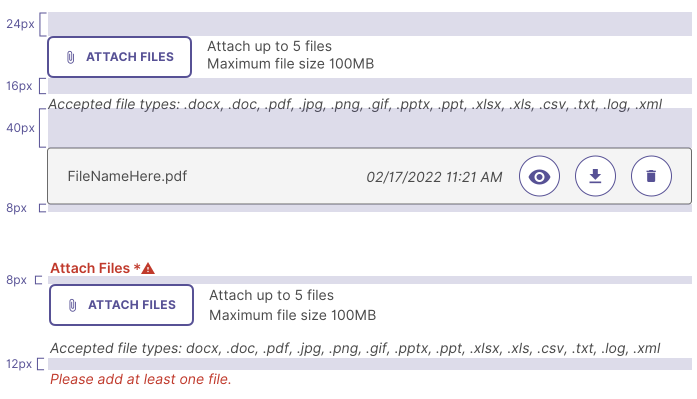

Tip: Keep 80px between the file name and date uploaded. File name will be truncated to fit this spacing.
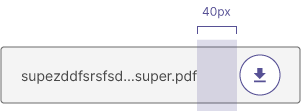
At less than 500px, the spacing in between the action button and file name is 40px so that the user sees more of the file name.
An ellipsis should be used for truncated characters and should represent three or more truncated characters in the file name (ex. thisisalon…ame.doc). There must be at least five characters of non-truncated content in the file name. On hover there should always be a tooltip that shows the entire non-truncated file name.